Authentication is handled using query parameters. This query parameter should be included in all of your API requests, and the value of this parameter should be your API token. All endpoints require authentication and require you to set the authorization header.
Using query parameters to authenticate requests is useful when you want to express a request entirely in a URL. This method is also referred as pre-signing a URL.
Creating an API Key
- Sign in to https://console.voice.virlow.com, and from the left menu select API Keys.
- Click on the plus icon to add a new API Key.
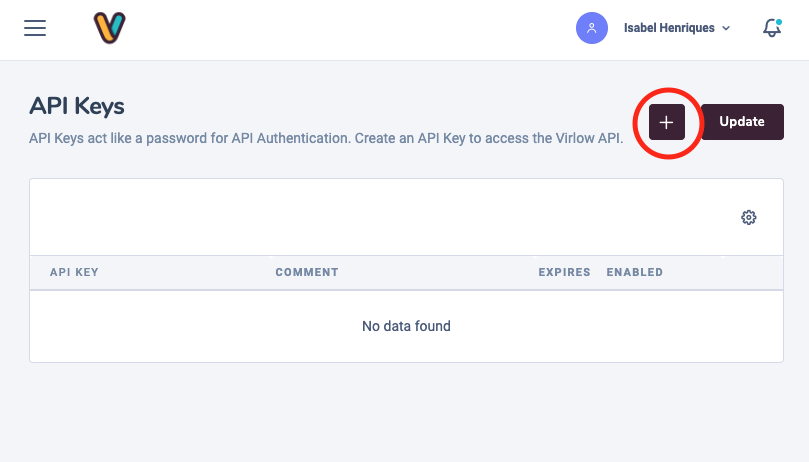
- Enter a Comment, change the Enabled status to either True or False, set an expiration date and click Add API Key.
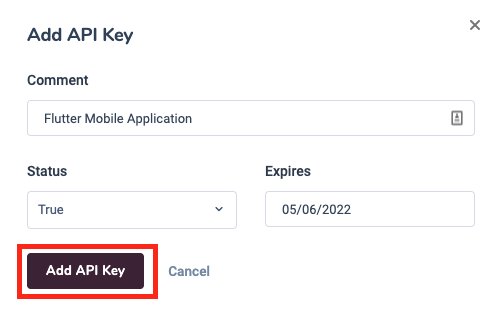
- You can now use your API key within your application.
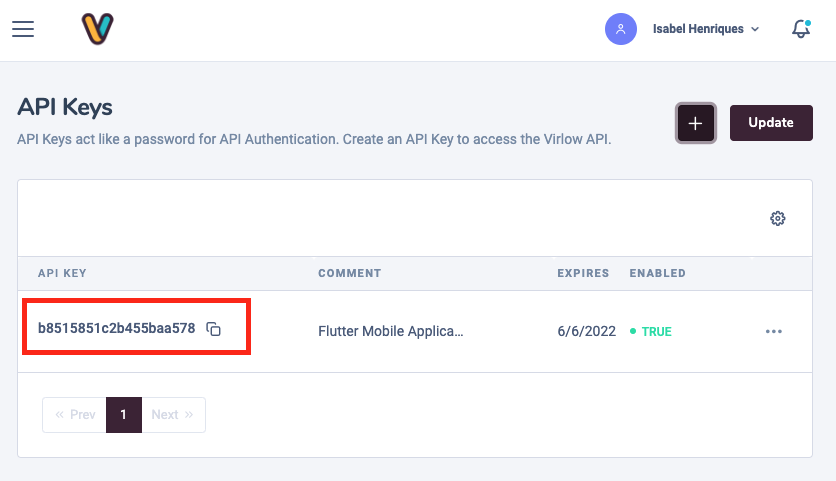
Asynchronous Transcription Authentication Example
You pass the API Key to our API using the x-api-key
parameter via the query string.
url: 'https://api.voice.virlow.com/v1-beta/transcript?x-api-key=YOUR_API_KEY'
var axios = require('axios');
var FormData = require('form-data');
var fs = require('fs');
var data = new FormData();
data.append('audio', fs.createReadStream('audio_1.wav'));
data.append('dual_channel', 'false');
data.append('punctuate', 'true');
data.append('webhook_url', '');
data.append('speaker_diarization', 'false');
data.append('language', 'enUs');
data.append('short_hand_notes', 'true');
data.append('tldr', 'true');
data.append('custom', 'YOUR_VALUE');
var config = {
method: 'post',
url: 'https://api.voice.virlow.com/v1-beta/transcript?x-api-key=YOUR_API_KEY',
headers: {
...data.getHeaders()
},
data : data
};
axios(config)
.then(function (response) {
console.log(JSON.stringify(response.data));
})
.catch(function (error) {
console.log(error);
});
Real-Time Streaming Transcription Authentication Example
Using the available Socket.io packages, pass your API Key to our API by using the x-api-key
parameter via the query string.
url: 'https://api.voice.virlow.com/v1-beta/transcript?x-api-key=YOUR_API_KEY'
const socketio = io("https://api.voice.virlow.com/v1-beta/transcript?x-api-key=YOUR_API_KEY", {
forceNew: true,
transports: ['polling']
});
socket = new Socket("http://api.falavoice.com?x-api-key=YOUR_API_KEY");